Here are the examples of the python api pexpect.TIMEOUT taken from open source projects. By voting up you can indicate which examples are most useful and appropriate. By voting up you can indicate which examples are most useful and appropriate. View license def socketfn(self, timedout, allread): result = 0 try: sock = socket.socket(socket.AFINET, socket.SOCKSTREAM) sock.connect((self.host, self.port)) session = fdpexpect.fdspawn(sock, timeout=10) # Get all data from server session.readnonblocking(size=4096) allread.set # This read should timeout session.readnonblocking(size=4096) except pexpect.TIMEOUT: timedout.set.
- Python Serial Timeout Example Documentation
- Python Serial Timeout Example Python
- Python Serial Timeout Example Sheet
- Python Serial Disable Timeout
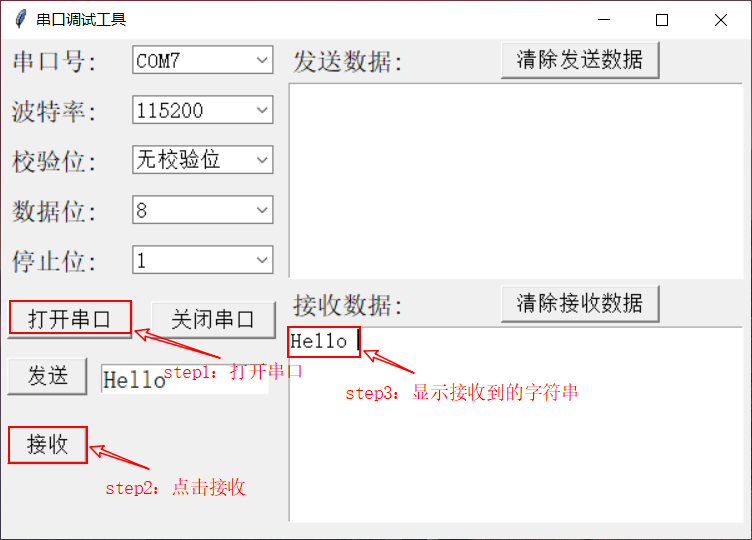
Bases: object
Read (length, timeout=None) source ¶ Read up to length number of bytes from the serial port with an optional timeout. Timeout can be positive for a timeout in seconds, 0 for a non-blocking read, or negative or None for a blocking read that will block until length number of bytes are read. Default is a blocking read. How to use the serial port. This port is visible in Linux as /dev/ttyUSB3 device. Example in Python. Install the Python serial library: sudo apt-get update sudo apt-get install python-serial This example sends a string on the serial port each 1 second at 115200,N,8,1 and checks if any character is received from a remote serial terminal.
Instantiate a Serial object and open the tty device at the specifiedpath with the specified baudrate, and the defaults of 8 data bits, noparity, 1 stop bit, no software flow control (xonxoff), and no hardwareflow control (rtscts).
Parameters: |
|
---|---|
Returns: | Serial object. |
Return type: | |
Raises: |
|
read
(length, timeout=None)[source]¶Read up to length number of bytes from the serial port with anoptional timeout.
timeout can be positive for a timeout in seconds, 0 for anon-blocking read, or negative or None for a blocking read that willblock until length number of bytes are read. Default is a blockingread.
For a non-blocking or timeout-bound read, read() may return data whoselength is less than or equal to the requested length.
Parameters: |
|
---|---|
Returns: | data read. |
Return type: | bytes |
Raises: |
|
write
(data)[source]¶Write data to the serial port and return the number of byteswritten.
Parameters: | data (bytes, bytearray, list) – a byte array or list of 8-bit integers to write. |
---|---|
Returns: | number of bytes written. |
Return type: | int |
Raises: |
|
poll
(timeout=None)[source]¶Poll for data available for reading from the serial port.
timeout can be positive for a timeout in seconds, 0 for anon-blocking poll, or negative or None for a blocking poll. Default isa blocking poll.
Parameters: | timeout (int, float, None) – timeout duration in seconds. |
---|---|
Returns: | True if data is available for reading from the serial port, False if not. |
Return type: | bool |
flush
()[source]¶Flush the write buffer of the serial port, blocking until all bytesare written.
Raises: | SerialError – if an I/O or OS error occurs. |
---|
input_waiting
()[source]¶Query the number of bytes waiting to be read from the serial port.
Returns: | number of bytes waiting to be read. |
---|---|
Return type: | int |
Raises: | SerialError – if an I/O or OS error occurs. |
output_waiting
()[source]¶Query the number of bytes waiting to be written to the serial port.
Returns: | number of bytes waiting to be written. |
---|---|
Return type: | int |
Raises: | SerialError – if an I/O or OS error occurs. |
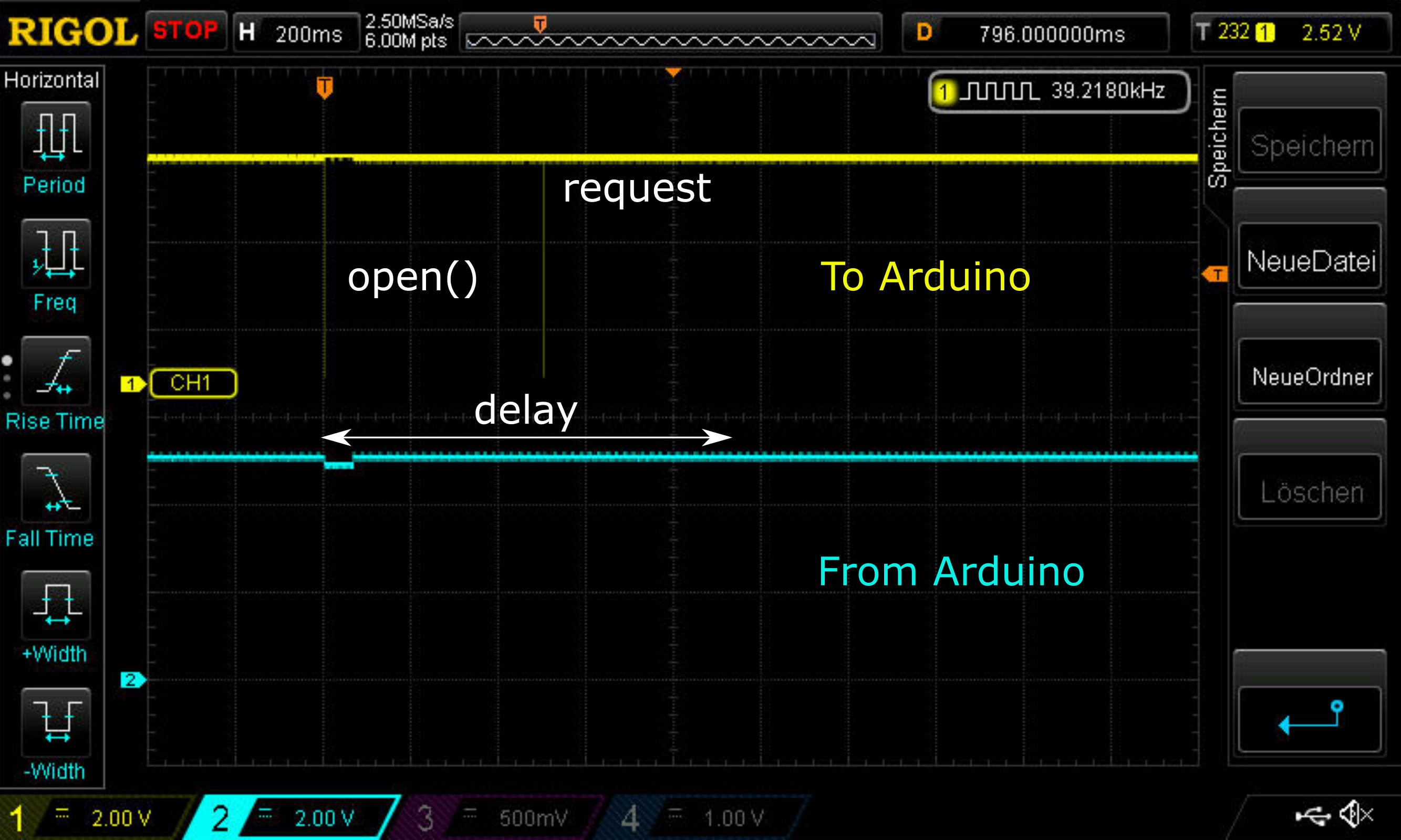
close
()[source]¶Close the tty device.
Raises: | SerialError – if an I/O or OS error occurs. |
---|
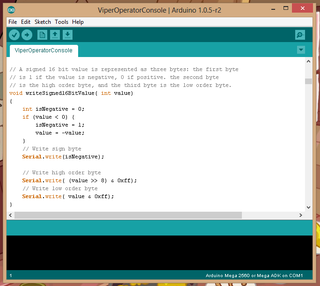
fd
¶Get the file descriptor of the underlying tty device.
Type: | int |
---|
Python Serial Timeout Example Documentation
devpath
¶Get the device path of the underlying tty device.

Type: | str |
---|
Python Serial Timeout Example Python
baudrate
¶Get or set the baudrate.
Raises: |
|
---|---|
Type: | int |
databits
¶Get or set the data bits. Can be 5, 6, 7, 8.
Raises: |
|
---|---|
Type: | int |
parity
¶Get or set the parity. Can be “none”, “even”, “odd”.
Raises: |
|
---|---|
Type: | str |
stopbits
¶Get or set the stop bits. Can be 1 or 2.
Raises: |
|
---|---|
Type: | int |
xonxoff
¶Get or set software flow control.
Raises: |
|
---|---|
Type: | bool |
rtscts
¶Get or set hardware flow control.
Raises: |
|
---|---|
Type: | bool |
Interfacing with a RS232 serial device is a common task when using Python in embedded applications. The easiest way to get python talking to serial ports is use the pyserial project found at http://pyserial.sourceforge.net/. This module works on most platforms and is straightforward to use (see examples on project web site). However, getting the read function in this module to operate in an optimal way takes a little study and thought. This article investigates how the pyserial module works, possible issues you might encounter, and how to optimize serial reads.
We start out with several goals as to how we want the application to behave in relation to the serial port:
- application must block while waiting for data.
- for performance reasons, we want to read decent size chunks of data at a time if possible. Python function calls are expensive, so performance will be best if we can read more than one byte at a time.
- We want any data received returned in a timely fashion.
A key parameter in the pyserial Serial class is the timeout parameter. This parameter is defined as:
The Serial class read function also accepts a size parameter that indicates how many characters should be read. Below is the source for the read function on Posix systems (Linux, etc):
The easy way to use this module is to simply set the timeout to None, and read size to 1. This will return any data received immediately. But, this setup is very inefficient when transferring large amounts of data due to the Python processing overhead.
To meet our goal of reading multi-byte blocks of data at a time, we need to pass the read function a size greater than 1. However, if timeout is set to None, the read will block until size bytes have been read, which does not meet the goal of returning any data read in a timely fashion. The solution then is to:
Python Serial Timeout Example Sheet
- set the read size high enough to get good performance
- set the timeout low enough so that any data received is returned in a reasonable timeframe, but yet the application spends most of its time blocked if there is no data.
Python Serial Disable Timeout
As an example, a size of 1000 and a timeout of 1 second seems to perform well. When used this way, the pyserial module performs well and returns all data read quickly.